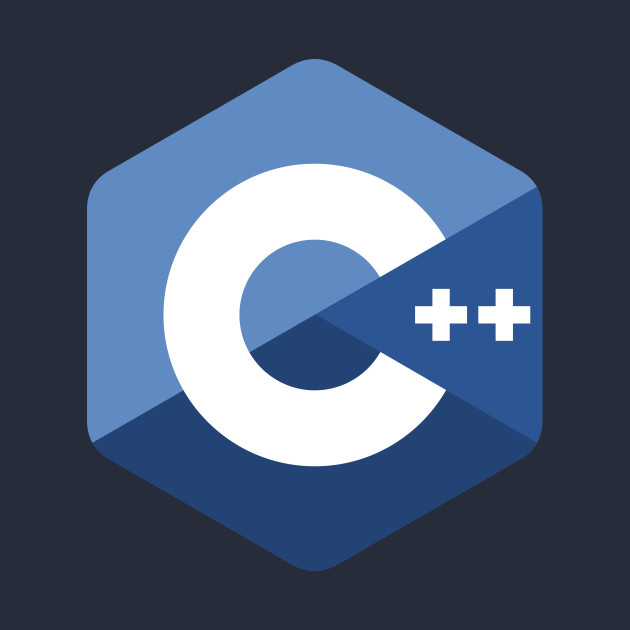
- Function Templates
- Function templates define a family of functions for different template arguments.(함수 템플릿은 다양한 템플릿 인자에 대한 함수군을 정의한다.)
- When you pass arguments to function paramters depending on template parameters, function templates deduce the template parameters to be instantiated for the corresponding parameter types.(템플릿 파라미터에 종속된 함수 파라미터에 인자를 전달하면, 함수 템플릿은 템플릿 파라미터를 연역한 후, 해당하는 타입으로 인스턴스화 된다.)
- You can explicitly qualify the leading template parameters.(템플릿 파라미터를 명시적으로 한정지을 수 있다.)
- You can define default arguments for template parameters. These may refer to previous template parameters and be followed by parameters not having default arguments.(템플릿 파라미터에 디폴트 인자를 정의할 수 있다. 그뒤에 디폴트 인자를 가지지 않는 파라미터가 나올 수도 있다.)
- You can overload function templates.(함수 템플릿은 오버로드할 수 있다.)
- When overloading function templates with other function templates, you should ensure that only one of them matches for any call.(함수 템플릿을 다른 함수 템플릿으로 오버로딩할 때, 하나의 호출은 하나의 함수 템플릿에 매치되야 된다.)
- When you overload function templates, limit your changes to specifying template parameters explicitly.(함수 템플릿을 오버로딩할 때, 템플릿 파라미터를 명시하는 것, 이상으로 수정하지 않는다.)
- Ensure the compiler sees all overloaded versions of function templates before you call them.(함수 템플릿을 호출하기 전에, 컴파일러가 오버로드된 모든 함수 템플릿을 볼 수 있도록 한다.)
- Class Templates
- A class template is a class that is implemented with one or more type parameters left open.(클래스 템플릿은 하나 이상의 타입 파라미터가 열린 상태로 구현된 클래스다.)
- To use a class template, you pass the open types as template arguments. The class template is then instantiated (and compiled) for these types.(클래스 템플릿을 사용할 때, 열린 타입을 템플릿 인자로 전달한다. 그후, 클래스 템플릿은 인스턴스화(그리고 컴파일)된다.)
- For class templates, only those member function that are called are instantiaed.(클래스 템플릿에서는, 호출된 멤버 함수만 인스턴스화 된다.)
- You can specialize class templates for certain types.(특정 타입에 대해서만, 클래스 템플릿을 특수화할 수 있다.)
- You can partially specialize class templates for certain types.(특정 타입에 대해서만, 부분 클래스 템플릿을 부분 특수화할 수 있다.)
- Since C++17, class template arguments can automatically be deduced from constructors.(C++17부터는 생성자로 클래스 템플릿 인자를 자동으로 연역할 수 있다.)
- You can define aggregate class templates.(어그리게이트 클래스 템플릿도 정의할 수 있다.)
- Call parameters of a template type decay if delared to be called by value.(템플릿 타입의 파라미터 호출은 call by value일 때만, 붕괴한다.)
- Templates can only be declared and defined in global/namespace scope or inside class declarations.(템플릿은 전역/네임스페이스 영역이나, 클래스 선언 안에서 선언되고 정의될 수 있다.)
- Nontype Template Parameters
- Templates can have parameters that are values rather than types.(템플릿은 타입보다는 값을 인자로 가질 수 있다.)
- You cannot use floating-point numbers or class-type objects as arguments for nontype template parameters. For pointers/references to string literals, temporaries, and subobjects, restrictions apply.(부동소수점 숫자나 클래스타입의 객체는 논타입 템플릿 인자로 쓸 수 없다. 문자열 리터럴, 임시객체, 그리고 하위객체들을 가리키는 포인터/레퍼런스는 제약사항이 적용된다.)
- Using auto enables templates to have nontype template parameters that are values of generic types.(auto를 사용하면 논타입 템플릿 인자가 제네릭 타입을 값으로 가질 수 있다.)
- Variadic Templates
- By using parameter packs, templates can be defined for an arbitary number of template parameters of arbitary type.
- To process the parameters, you need recursion and/or a matching novariadic function.
- Operator sizeof... yields the number of arguments provided for a parameter pack.
- A typical application of variadic templates is fowarding an arbitary number of arguments of arbitary type.
- By using fold expressions, you can apply operator to all arguments of paramter pack.
- Basics
- To access a type name that depends on a template parameter, you have to qualify the name with a leading typename.
- To access members of bases classes that depends on template parameters, you have to qualify the access by this-> or their class name.
- Nested classes and member functions can also be templates. One application is the ability to implements generic operations with internal type conversions.
- Template version of constructors or assignment operators don't replace predefined constructors or assignment operators.
- By using braced initialization or explicitly calling a default constructor, you can ensure that variables and member of templates are initialized with a default value even if they are instantiated with a built-in type.
- You can provide specific templates for raw arrays, which can also be applicable to string literals.
- When passing raw arrays or string literals, arguments decay (perform an array-to-pointer conversion) during argument deduction if and only if the parameter is not a reference.
- You can define variable templates(since C++14).
- You can also use class templates as template parameters, as template template parameters.
- Template template arguments must ususally match their parameters exactly.
- Move Semantics and enable_if<>
- In templates, you can “perfectly” forward parameters by declaring them as forwarding references (declared with a type formed with the name of a template parameter followed by &&) and using std::forward<>() in the forwarded call.
- When using perfect forwarding member function templates, they might match better than the predefined special member function to copy or move objects.
- With std::enable_if<>, you can disable a function template when a compile-time condition is false (the template is then ignored once that condition has been determined).
- By using std::enable_if<> you can avoid problems when constructor templates or assignment operator templates that can be called for single arguments are a better match than implicitly generated special member functions.
- can templify (and apply enable_if<>) to special member functions by deleting the predefined special member functions for const volatile.
- Concepts will allow us to use a more intuitive syntax for requirements on function templates.
- By value and By reference.
- When testing templates, use string literals of different length.
- Template parameters passed by value decay, while passing them by reference does not decay.
- The type trait std::decay<> allows you to decay parameters in templates passed by reference.
- In some cases std::cref() and std::ref() allow you to pass arguments by reference when function templates declare them to be passed by value.
- Passing template parameters by value is simple but may not result in the best performance.
- Pass parameters to function templates by value unless there are good reasons to do otherwise.
- Ensure that return values are usually passed by value (which might mean that a template parameter can’t be specified directly as a return type).
- Always measure performance when it is important. Do not rely on intuition; it’s probably wrong.
- Compile-Time Programming
- Templates provide the ability to compute at compile time (using recursion to iterate and partial specialization or operator ?: for selections).
- ith constexpr functions, we can replace most compile-time computations with “ordinary functions” that are callable in compile-time contexts.
- With partial specialization, we can choose between different implementations of class templates based on certain compile-time constraints.
- Templates are used only if needed and substitutions in function template declarations do not result in invalid code. This principle is called SFINAE (substitution failure is not an error).
- SFINAE can be used to provide function templates only for certain types and/or constraints.
- nce C++17, a compile-time if allows us to enable or discard statements according to compile-time conditions (even outside templates).
- Basic Templates
- Use class template, function template, and variable template for classes, functions, and variables, respectively, that are templates.
- Template instantiation is the process of creating regular classes or functions by replacing template parameters with concrete arguments. The resulting entity is a specialization.
- Types can be complete or incomplete.
- According to the one-definition rule (ODR), noninline functions, member functions, global variables, and static data members should be defined only once across the whole program.
- Gneric Libraries
- Templates allow you to pass functions, function pointers, function objects, functors, and lambdas as callables.
- When defining classes with an overloaded operator(), declare it as const (unless the call changes its state).
- With std::invoke(), you can implement code that can handle all callables, including member functions.
- Use decltype(auto) to forward a return value perfectly.
- Type traits are type functions that check for properties and capabilities of types.
- Use std::addressof() when you need the address of an object in a template.
- Use std::declval() to create values of specific types in unevaluated expressions.
- Use auto&& to perfectly forward objects in generic code if their type does not depend on template parameters
- Be prepared to deal with the side effects of template parameters being references.
- You can use templates to defer the evaluation of expressions (e.g., to support using incomplete types in class templates).
'C++' 카테고리의 다른 글
값 카테고리, 타입 카테고리 (0) | 2022.07.04 |
---|---|
Type Category Testing_2(형 카 검-컴포지트 타입) (0) | 2022.07.04 |
Type Category Testing_1(형 카 검-내장타입) (0) | 2022.07.03 |
[C++] Return Value Optimization(RVO) (0) | 2021.01.04 |
std::exception과 Stack Unwinding 과 RAII (0) | 2020.12.04 |