[LeetCode] Maximum Subarray - Kadane Algorithm
2022. 9. 21. 12:03
Problem set
Maximum Subarray - LeetCode Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com int maxSubArray(vector& nums){ int currentSum = 0; int bestSum = INT_MIN; for(auto& ele : nums){ currentSum = std::max(ele, currentSum + ele); if(currentSum > bestSum){ bestSum = currentSum; } } return bestSum; }
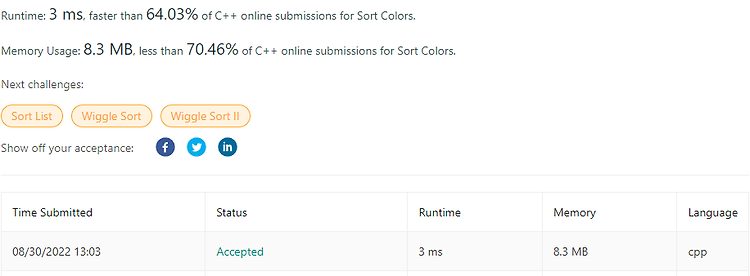
[LeetCode] Sort Colors
2022. 8. 30. 13:14
Problem set
Sort Colors - LeetCode Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com void sortColors(vector& nums){ int pivovIndex = 0; int currentColor = 0; while(pivovIndex < nums.size()){ for(int i = pivovIndex; i < nums.size(); i++){ if(nums[i] == currentColor){ std::swap(nums[pivovIndex], nums[i]); ..
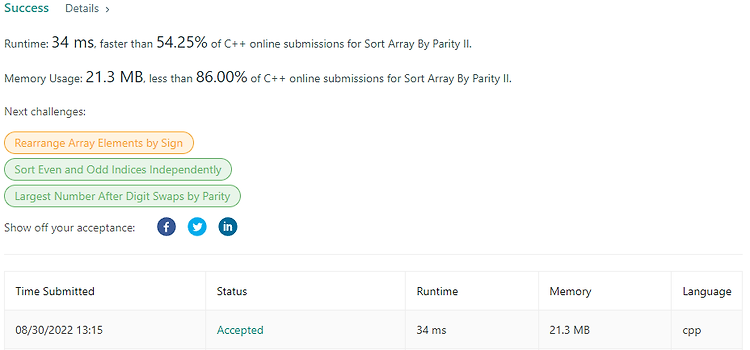
[LeetCode] Sort-Array-By-Parity-ii
2022. 8. 26. 13:28
Problem set
Sort Array By Parity II - LeetCode Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com std::vector sortArrayByParityII(std::vector& nums){ int even_index = 0; int odd_index = 1; std::vector res; res.resize(nums.size()); for(int i = 0; i < nums.size(); i++){ if(nums[i] % 2 == 0){ res[even_index]..
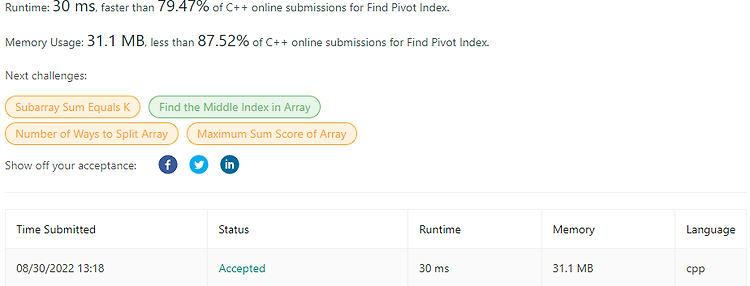
[LeetCode] Find Pivot Index
2022. 8. 26. 10:05
Problem set
Find Pivot Index - LeetCode Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com int pivotIndex(vector& nums){ int left_sum = 0; int right_sum = std::accumulate(nums.begin(), nums.end(), 0); int current_left = 0; for(int i = 0; i < nums.size(); i++){ left_sum += current_left; right_sum -= nums[i..
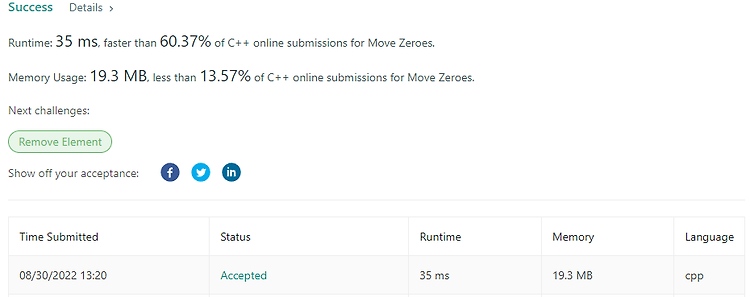
[LeetCode] Move Zeroes
2022. 8. 26. 09:32
Problem set
Move Zeroes - LeetCode Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com void moveZeroes(vector& nums){ int wideIndex = 0; for(int i = 0; i < nums.size(); i++){ if(nums[i] != 0){ swap(nums[i], nums[wideIndex]); wideIndex++; } } }
[백준] 1967 트리의 지름
2021. 2. 20. 21:05
Problem set
1967번: 트리의 지름 파일의 첫 번째 줄은 노드의 개수 n(1 ≤ n ≤ 10,000)이다. 둘째 줄부터 n-1개의 줄에 각 간선에 대한 정보가 들어온다. 간선에 대한 정보는 세 개의 정수로 이루어져 있다. 첫 번째 정수는 간선이 연 www.acmicpc.net #include #include #include #include using namespace std; struct Edge { int to; int cost; Edge(int to, int cost) : to(to), cost(cost) { } }; vector a[10001]; bool check[10001]; int dist[10001]; void bfs(int start) { memset(dist,0,sizeof(dist)); memse..
[백준] 1167 트리의 지름
2021. 2. 20. 21:03
Problem set
1167번: 트리의 지름 트리가 입력으로 주어진다. 먼저 첫 번째 줄에서는 트리의 정점의 개수 V가 주어지고 (2≤V≤100,000)둘째 줄부터 V개의 줄에 걸쳐 간선의 정보가 다음과 같이 주어진다. (정점 번호는 1부터 V까지 www.acmicpc.net #include #include #include #include using namespace std; struct Edge { int to; int cost; Edge(int to, int cost) : to(to), cost(cost) { } }; vector a[100001]; bool check[100001]; int dist[100001]; void bfs(int start) { memset(dist,0,sizeof(dist)); memset(..
[백준] 11725 트리의 부모 찾기
2021. 2. 20. 21:00
Problem set
11725번: 트리의 부모 찾기 루트 없는 트리가 주어진다. 이때, 트리의 루트를 1이라고 정했을 때, 각 노드의 부모를 구하는 프로그램을 작성하시오. www.acmicpc.net #include #include #include #include #include #include #include #include #include using namespace std; const int MAX = 100111; vector a[MAX]; int parent[MAX]; bool check[MAX]; int depth[MAX]; int dist[MAX]; int main() { int n; scanf("%d",&n); for (int i=0; i