[LeetCode] Maximum Subarray - Kadane Algorithm
2022. 9. 21. 12:03
Problem set
Maximum Subarray - LeetCode Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com int maxSubArray(vector& nums){ int currentSum = 0; int bestSum = INT_MIN; for(auto& ele : nums){ currentSum = std::max(ele, currentSum + ele); if(currentSum > bestSum){ bestSum = currentSum; } } return bestSum; }
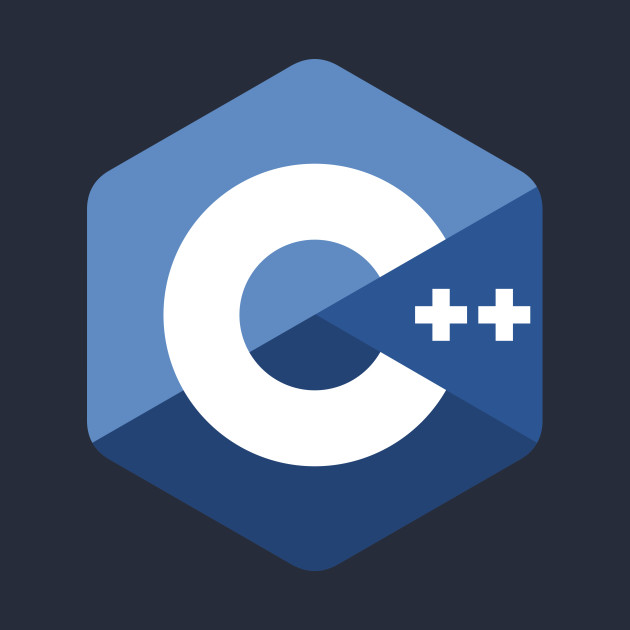
[C++] Iterator, 반복자
2022. 9. 19. 12:39
C++
[C++] 범위기반 for 루프를 위한 클래스 템플릿 최소 구현 반복자를 증가시키는 operator++ 반복자를 역참조하고 반복자가 가리키는 값에 접근하는 operator* 다른 반복자와 비항등성을 비교하는 operator!= begin(), end() 함수 #include #include template class dummy_a.. entrypoint.tistory.com 이전에 이터레이터를 구현하는 글을 작성했었다. 이터레이터란 무엇이냐?, 이터레이터는 STL 컨테이너를 다루기 위한 일반화된 포인터이다. 이터레이터라는 개념없이, STL 컨테이너를 구현할 수 없다. 즉, 이터레이터는 곧 컨테이너이다. STL의 이터레이터는 기본적으로 다음의 연산을 지원한다. * 원소의 현재 위치를 반환한다. ==, !=..
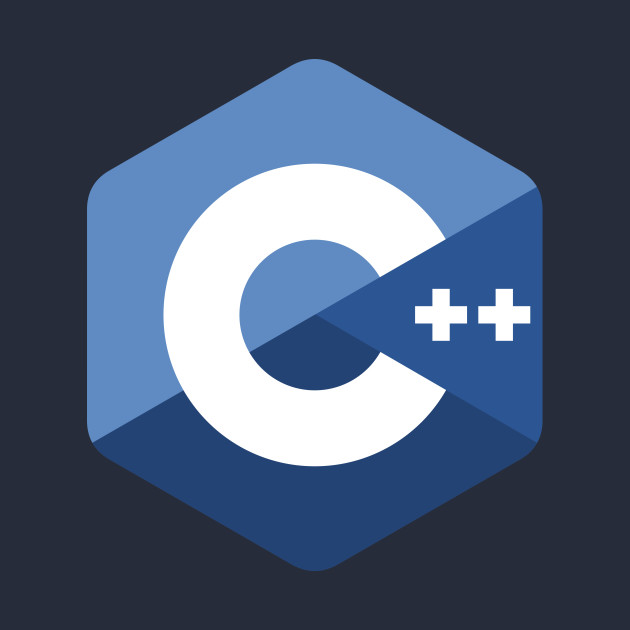
[C++14] Virtual Inheritance
2022. 9. 18. 14:32
C++
다이아몬드 상속의 문제점은 상속의 모호성이다. 부모 클래스가 상속하는 메서드들이 중복된다면, 컴파일 에러를 일으킨다. 이에 대한 해결방안으로는 중복된 메서드를 제거함으로써 모호성을 제거하는 것이었지만, virtual 상속을 이용한다면, 메서드를 제거하지 않고도, 모호성 문제를 해결할 수 있다. class Hero{ public: virtual void SpecialMove() = 0; virtual void Attack(){ cout
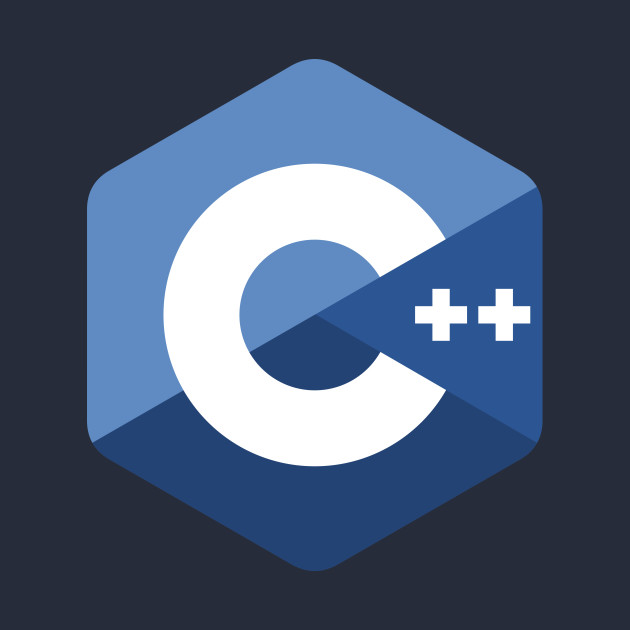
[C++20] Concepts
2022. 9. 17. 19:13
C++
C++20에는 여러가지 개선사항이 추가되었다. 그중에서 concept를 소개하고자한다. 하지만 먼저, concept가 나온 배경이 무엇이지 알아보자. C++에는 Narrowing Conversion과 Integral Promotion이 있다. 데이터 전달과정에서 double이나 float형의 변수가 int형으로 Conversion이 일어나면, accuracy에서 손해를 봐야했으며, 반대로 bool 타입의 변수가 int로 Promotion이 일어나기도 했다. 이러한 문제로 인해서, 함수 템플릿을 작성할 때, 특정 타입에 대해서, 하나하나 Specilization 함수를 작성해야 했다. std::sort는 정렬 알고리즘이며, random access iterator를 사용하여 컨테이너의 원소들을 정렬한다. ..
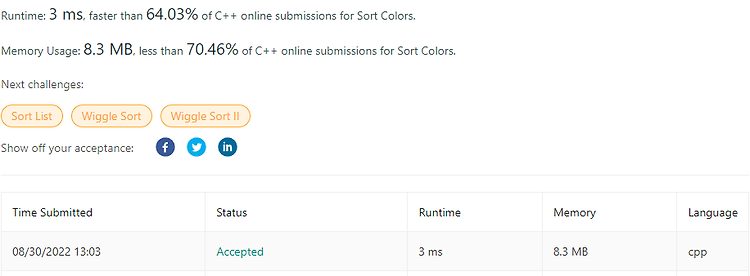
[LeetCode] Sort Colors
2022. 8. 30. 13:14
Problem set
Sort Colors - LeetCode Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com void sortColors(vector& nums){ int pivovIndex = 0; int currentColor = 0; while(pivovIndex < nums.size()){ for(int i = pivovIndex; i < nums.size(); i++){ if(nums[i] == currentColor){ std::swap(nums[pivovIndex], nums[i]); ..
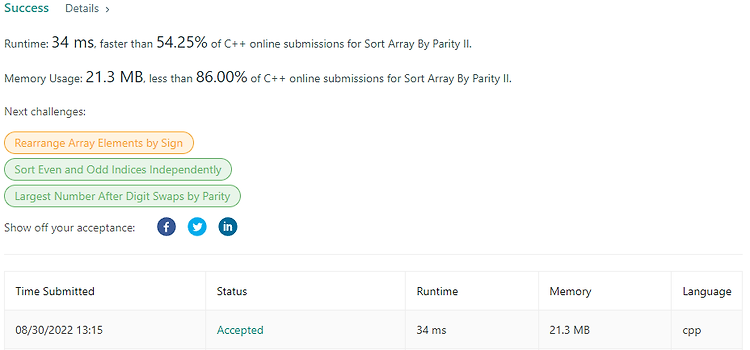
[LeetCode] Sort-Array-By-Parity-ii
2022. 8. 26. 13:28
Problem set
Sort Array By Parity II - LeetCode Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com std::vector sortArrayByParityII(std::vector& nums){ int even_index = 0; int odd_index = 1; std::vector res; res.resize(nums.size()); for(int i = 0; i < nums.size(); i++){ if(nums[i] % 2 == 0){ res[even_index]..
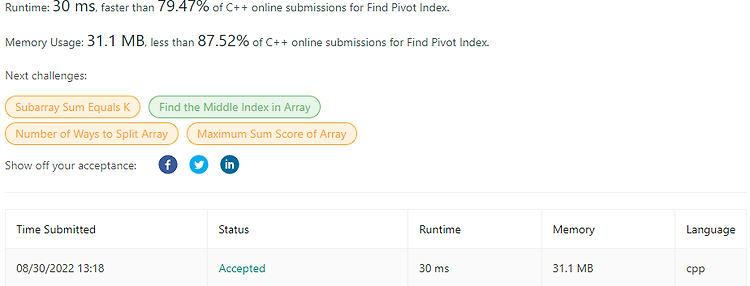
[LeetCode] Find Pivot Index
2022. 8. 26. 10:05
Problem set
Find Pivot Index - LeetCode Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com int pivotIndex(vector& nums){ int left_sum = 0; int right_sum = std::accumulate(nums.begin(), nums.end(), 0); int current_left = 0; for(int i = 0; i < nums.size(); i++){ left_sum += current_left; right_sum -= nums[i..
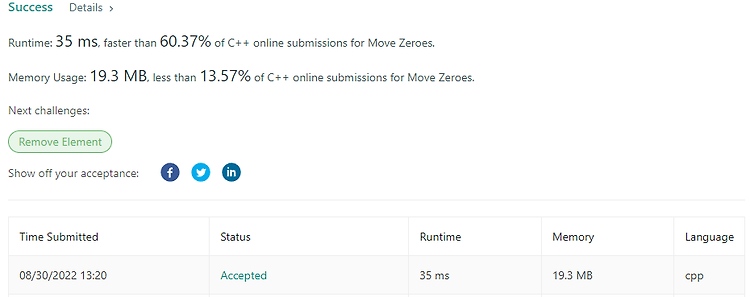
[LeetCode] Move Zeroes
2022. 8. 26. 09:32
Problem set
Move Zeroes - LeetCode Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com void moveZeroes(vector& nums){ int wideIndex = 0; for(int i = 0; i < nums.size(); i++){ if(nums[i] != 0){ swap(nums[i], nums[wideIndex]); wideIndex++; } } }